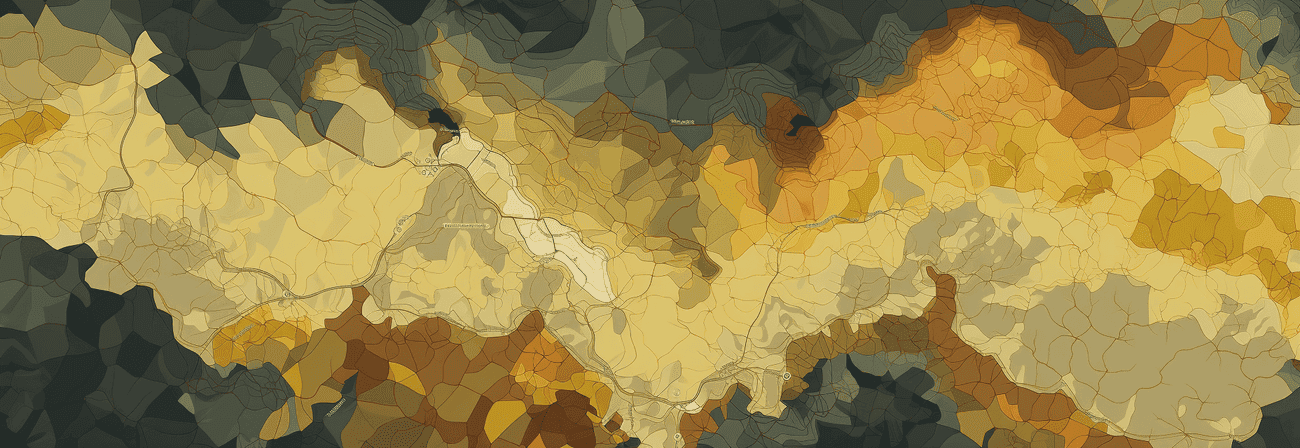
Export QGIS layers as images with PyQGIS
PyQGIS is a powerful tool that enables the automation of various processes, including the seamless export of images for all layers from a map.
To start the automation, you'll need one or more layers containing raster and/or vector data.
Add layers with PyQGIS
In the initial step, if all your files reside in the same folder, you can use a "for .. in loop" to read them in. By adding .endswith(".gpkg")
, for instance, you can specifically target files with the ".gpkg" extension. The layer names are then stored in an array for future reference.
import os, sys from PyQt5.QtCore import QTimer # path to look for files path = "ordner/nocheinordner/" # set path dirs = os.listdir( path ) # array for storing layer_names layer_list = [] # variable for further processing count = 0 #look for files inpath for file in dirs: # search for ".gpkg" files if file.endswith(".gpkg"): #add vectorlayers vlayer = iface.addVectorLayer(path + file, "Layername", "ogr") layer_list.append(vlayer.name())
The newly added vector layers will then appear in the QGIS layer tree.
Image Export for Each Layer
Once you are satisfied with the display, you can use two functions to export a georeferenced image for each layer.
def prepareMap(): # make all layers invisible iface.actionHideAllLayers().trigger() # get layer by layer_name layer_name = QgsProject.instance().mapLayersByName(layer_list[count])[0] # select layer iface.layerTreeView().setCurrentLayer(layer_name) # set selected layer visible iface.actionShowSelectedLayers().trigger() # Wait a second and export the map QTimer.singleShot(1000, exportMap)
The "prepareMap ()" function first deactivates all layers. A layer is then selected from the "layer_list" array using its layer name and then displayed again. The QTimer class is particularly important here. Before an image is created, there must always be a short wait before the selected layer is really visible. Without QTimer, the script would run so quickly that the result would be loud images with the same content. After waiting a second, the "exportMap" function is called.
def exportMap(): global count # save current view as image iface.mapCanvas().saveAsImage( path + layer_list[count] + ".png" ) # feedback for printed map print('{}.png exported sucessfully'.format(layer_list[count])) # get map for every layer in layer_list if count < len(layer_list)-1: # Wait a second and prepare next map (timer is needed because otherwise all images have the samec content # the script excecutes faster then the mapCanvas can be reloaded QTimer.singleShot(1000, prepareMap) count += 1
Now the current map, in which only one level is shown, is saved as a PNG image in the source directory. Ultimately, you "land" in a loop that goes through all the layers that are in the "layer_list" array and calls the "prepareMap" function for each layer again.
Table of contents
First published August 30, 2020
Have you published a response to this? Send me a webmention by letting me know the URL.
Found no Webmentions yet. Be the first!
About The Author

Geospatial Developer
Hi, I'm Max (he/him). I am a geospatial developer, author and cyclist from Rosenheim, Germany. Support me